JavaScript forEach | Looping Through an Array in JS
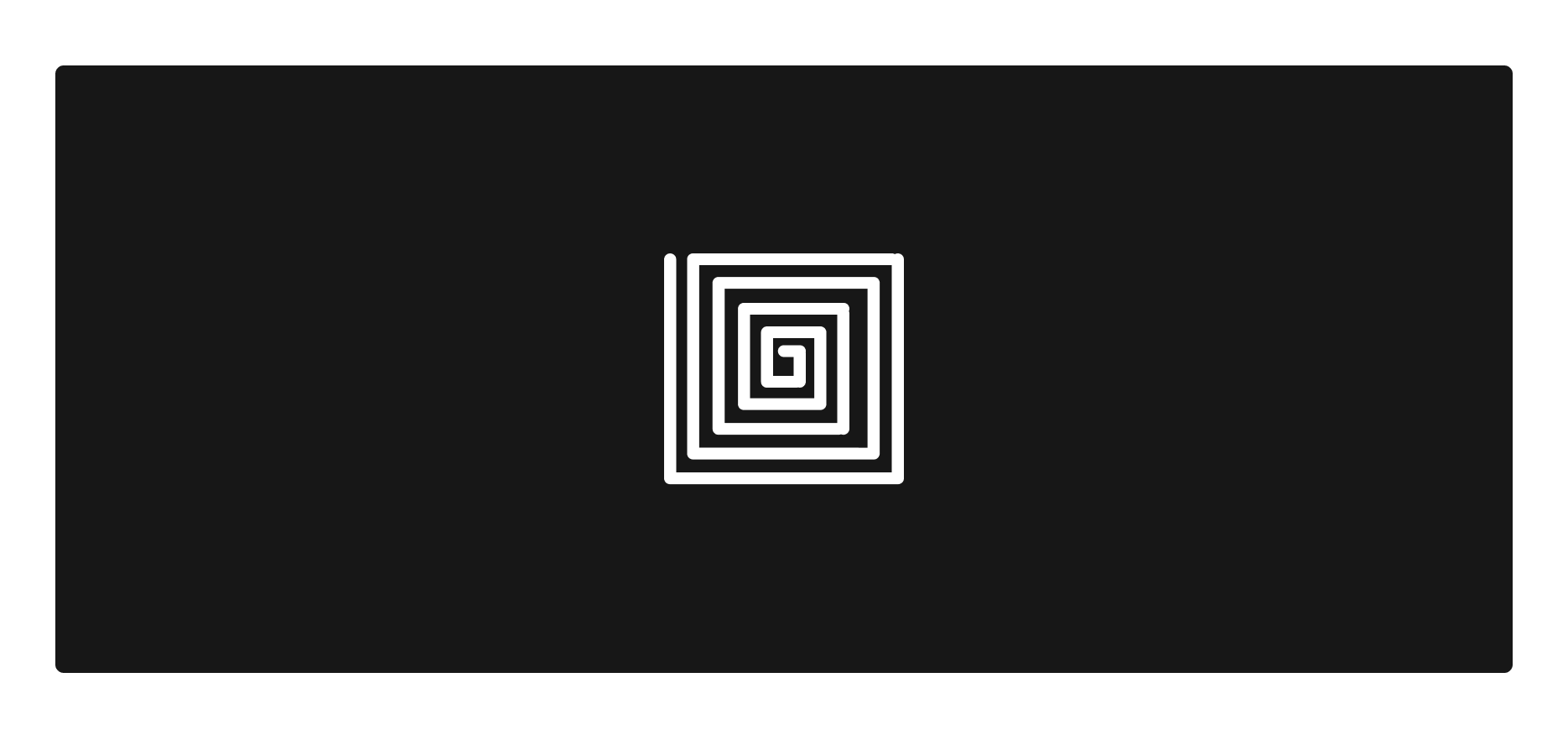
For many developers, JavaScript acts as introduction to the functional programming paradigm. And if you've never encountered callback functions before, the JavaScript for each function might look a little funny.
In this quick article, we're going to see how to iterate over an array of data using JavaScript's forEach function. We'll look at different ways to call it and discuss when to use it vs. the traditional for loop.
Using the JavaScript for each function
In JavaScript, the array object contains a forEach
method. This means that on any array, we can call forEach
like so:
let fruits = ['apples', 'oranges', 'bananas'];
fruits.forEach(function (item, index) {
console.log(item, index)
})
This should print out the following.
apples 0
oranges 1
bananas 2
Let's explain how this works.
- We pass an anonymous function to the
forEach
method. - Within that anonymous function, we include parameters to get access to the current
item
and the numericalindex
in the array that the item represents.
The function we provide to the forEach
function has three parameters (two of which are optional).
- (Required) The current element - Represents the current element
- (Optional) Index - Returns the current index
- (Optional) Array - Returns the entire array for each loop
Alternate ways to call it
Option 1: An anonymous function
The first of the alternate way to call this function is to utilize the anonymous function. This is what we just saw.
arr.forEach(function (item) { // Anonymous function
// Write what you'd like to happen here
})
Option 2: Using arrow functions
The second option is to utilize an arrow function.
arr.forEach((item, index) => console.log(item, index))
Try this out. It allows you to write the loop on a single line.
Option 3: A callback function
The last option is to utilize a callback function, which is really just a function defined separately that we pass in as an argument to the forEach
function.
let fruits = ['apples', 'oranges', 'bananas'];
function printPretty (item, index) {
console.log(`${index} - ${item}`)
}
fruits.forEach(printPretty);
This should print out the following:
0 - apples
1 - oranges
2 - bananas
When to use the for loop vs. a for loop
Most of us first learn about loops the traditional way - you know, the loops with counters that get incremented? Like this:
let fruits = ['apples', 'oranges', 'bananas'];
for (let index = 0; i < fruits.length; i++) {
console.log(fruits[i]);
}
The truth is, you can use either traditional for loops or forEach
loops. It doesn't matter.
However, using forEach
loops results in code that looks a lot more declarative and easy to read, especially if you're dealing with nested loops (which I opt to try to avoid in the first place).
Stay in touch!
Join 15000+ value-creating Software Essentialists getting actionable advice on how to master what matters each week. 🖖
View more in Web Development